In-depth look at interstitials
In-depth look: in-app interstitial
Let’s take a closer look at how the interstitial is created in this example from the main Interstitial documentation. Assuming your app is a game, where the method onLevelCompleted()
is called when the user completes a level:
public void onLevelCompleted() {
loadNextLevel();
}
Now instead of going to the next level, we’d like to show the interstitial first. Here is the example code again:
private InterstitialBuilder interstitialBuilder;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
// Preload the AppBrain interstitial. Settings the AdId is optional,
// but highly recommended. You can also create a custom AdId for your
// publisher app on our dashboard under "Ad settings".
interstitialBuilder = InterstitialBuilder.create()
.setAdId(AdId.LEVEL_COMPLETE)
.setOnDoneCallback(new Runnable() {
@Override
public void run() {
// Preload again, so we can use interstitialBuilder again.
interstitialBuilder.preload(getContext());
loadNextLevel();
})
.preload(this);
}
public void onLevelCompleted() {
interstitialBuilder.show(this);
}
As you can see, we first create an InterstialBuilder
instance using InterstitialBuilder.create()
. The InterstitialBuilder
has several options you can set, as described in the InterstitialBuilder javadoc. In this example two options are set. The first one is:
setAdId(AdId.LEVEL_COMPLETE)
Here we assign an AdId
to the interstitial we are creating. These are used to keep track of different interstitials in your app. AdId.LEVEL_COMPLETE
means this is the interstitial you show when the user has completed a level in the game. The AdId
class has several more predefined IDs you can use. You don’t have to set an AdId
when creating an interstitial, but it is recommended. For more information, see the AdId javadoc.
Secondly in our example, we call setOnDoneCallback()
. The InterstitialBuilder
is designed to have its methods “chained” together, so you can simply put setOnDoneCallback()
right behind another method call:
setAdId(AdId.LEVEL_COMPLETE).setOnDoneCallback(new Runnable() {
...
The method setOnDoneCallback()
takes a Runnable
as argument, so we create one. Alternatively you can use InterstitialBuilder.setListener()
to define an InterstitialListener
. With InterstitialListener
you can listen for more fine grained events from the interstitial. setListener()
and setOnDoneCallback()
can also be used at the same time.
After setting the ad ID and callback, we call preload()
on the InterstitialBuilder
. This will start loading the interstitial in the background, and, if an InterstitialListener
is set, will result in a callback to either onAdLoaded()
or onAdFailedToLoad()
.
If the interstitial successfully loads, it is ready to be shown, which is done by calling show()
, or alternatively maybeShow()
. The difference is that show()
will always try to show the interstitial, and will only return false
if something is wrong. maybeShow()
will only show the interstitial once in a while, determined by the AppBrain SDK. You can use this to prevent the user from seeing the interstitial too often.
In-depth look: interstitial on app exit
Another example from the Interstitial page shows how to show an interstitial when the user leaves your app:
private InterstitialBuilder interstitialBuilder;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
interstitialBuilder = InterstitialBuilder.create().setAdId(AdId.EXIT)
.setFinishOnExit(this).preload(this);
}
@Override
public void onBackPressed() {
if (!interstitialBuilder.show(this)) {
super.onBackPressed();
}
}
As you can see, we once again create an InterstitialBuilder
and set an AdId
, this time AdId.EXIT
. Now if the app also has an in-app interstitial, you can differentiate between the two on the AppBrain developer dashboard.
In this example we also call setFinishOnExit()
on the InterstitialBuilder
. We assume the method is called from an Activity
, so this
refers to the Activity
. Calling setFinishOnExit()
will make the Activity
close when the interstitial is closed.
Then we call preload()
, still in onCreate()
, so that we can call show()
in onBackPressed()
. The method show()
will return false
if for some reason the interstitial could not be displayed (e.g. the user currently has no internet connection). In that case we want to just close the app, so in the example we call super.onBackPressed()
, to let the Activity
handle the back press as normal.
Opening the offerwall directly
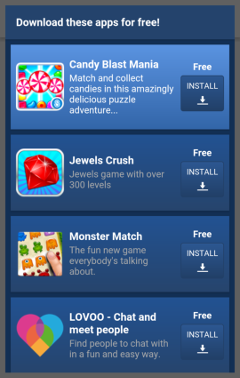
The default interstitial leads to the offerwall. In case you don’t want to use the interstitial, the AppBrain SDK can set the OnClickListener
of a View
for you so that it opens the offerwall directly:
AdService ads = AppBrain.getAds();
Button button = new Button(context);
button.setText(ads.getOfferWallButtonLabel(context));
ads.setOfferWallClickListener(context, button);
A similar method is available for opening the offerwall from a menu item:
@Override
public boolean onCreateOptionsMenu(Menu menu) {
AdService ads = AppBrain.getAds();
MenuItem item = menu.add(ads.getOfferWallButtonLabel(this));
ads.setOfferWallMenuItemClickListener(this, item);
return super.onCreateOptionsMenu(menu);
}
The above methods can be used without approval.
It is also possible to show the offerwall directly via AppBrain.getAds().showOfferWall()
. However, for using this method you need to request approval. Using showOfferWall() without approval won’t generate any revenue.
In our experience, the use of the interstitial gives up to 10x more revenue than other simple integrations using the offerwall directly. If you do feel you have a need to integrate without using the interstitial, please contact us at contact@appbrain.com to discuss this.