Interstitial
Showing the interstitial
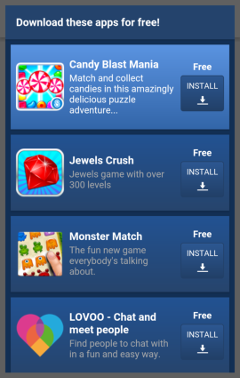
The AppBrain interstitial is the main way to generate revenue with the AppBrain SDK. The interstitial will show your users a list of apps they can install for free. Each app a user installs from the interstitial will generate revenue for you. Occasionally the SDK will show a pre-interstitial where the user is first asked if he’s interested in downloading interesting apps.
The best moment to show the interstitial is at a natural pause moment in your app, for instance when an important event just has completed, such as sending a photo or finishing a game level, or when leaving the app.
The easiest way to create an interstitial is using the InterstitialBuilder class. We recommend creating the InterstitialBuilder
in your activity’s onCreate()
, so it can start loading in the background. Then, for example in a view’s OnClickListener
, you can show the interstitial using InterstitialBuilder.show()
.
Instead of calling show()
you can also call maybeShow()
. When using that method, the AppBrain SDK automatically makes sure the interstitial is only shown occasionally, which is why maybe
is in the method name. Therefore it’s safe to call this method in multiple places without having to do your own rate limiting.
In-app interstitial
If you want a specific action to happen after a user closes the interstitial, you can set an onDoneCallback
in InterstitialBuilder
. This callback is always called after you try to show the interstitial, also when the interstitial fails to load, so you can safely put the next step of your application flow in there.
Here is an example of how to show the interstitial in a game at the end of a level, and start the next level when the user closes the interstitial:
private InterstitialBuilder interstitialBuilder;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
// Preload the AppBrain interstitial. Settings the AdId is optional,
// but highly recommended. You can also create a custom AdId for your
// publisher app on our dashboard under "Ad settings".
interstitialBuilder = InterstitialBuilder.create()
.setAdId(AdId.LEVEL_COMPLETE)
.setOnDoneCallback(new Runnable() {
@Override
public void run() {
// Preload again, so we can use interstitialBuilder again.
interstitialBuilder.preload(getContext());
loadNextLevel();
})
.preload(this);
}
public void onLevelCompleted() {
interstitialBuilder.show(this);
}
To get a more detailed look at this example, see In-depth look at interstitials.
Interstitial on app exit
To show the interstitial when the user leaves your Activity
, first create an InterstitialBuilder
in onCreate()
:
private InterstitialBuilder interstitialBuilder;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
interstitialBuilder = InterstitialBuilder.create().setAdId(AdId.EXIT)
.setFinishOnExit(this).preload(this);
}
Then, you can show the interstitial when the back button is pressed:
@Override
public void onBackPressed() {
if (!interstitialBuilder.show(this)) {
super.onBackPressed();
}
}
Note however, that if your activity puts fragments on the FragmentManager
back stack, you should make sure the back stack is empty before showing the interstitial:
@Override
public void onBackPressed() {
if (getFragmentManager().getBackStackEntryCount() > 0
|| !interstitialBuilder.show(this)) {
super.onBackPressed();
}
}
This examples and more options are discussed in detail on the In-depth look at interstitials page.