Integration with Unity
This section describes how to integrate the AppBrain SDK into your Unity app and start monetizing it.
Unity integration steps
Download
appbrain-unity.zip
from the AppBrain SDK GitHub pageUnzip
appbrain-unity.zip
to your Unity project’s Assets folder, e.g. for “MyProject” to:<MyProject>/Assets/
Open Unity with your project and call AppBrain functions where appropriate
To make integration even easier, the AppBrain SDK for Unity comes bundled with some scripts for common use cases. The scripts are located in <MyProject>/Assets/Plugins/Android/AppBrain/Scripts
.
AppBrain interstitial Unity script
The recommended way to use AppBrain in a game is to show the AppBrain interstitial when the player completes a level. You can use the script LevelCompleteInterstitial.cs
to do this. The class LevelCompleteInterstitial
is meant to be extended so you can override its OnNextLevel()
method. LevelCompleteInterstitial
itself extends Unity’s MonoBehaviour
class, so it can easily be used by any game object.
For example, you can add the following script to one of your game’s main objects:
public class MyInterstitial : AppBrainSdk.LevelCompleteInterstitial
{
protected override void OnNextLevel()
{
// TODO: Add logic to proceed to the next level.
}
}
You can then add a call to MyInterstitial.OnLevelComplete()
where appropriate in your game. This call will result in the AppBrain interstitial being shown. When the interstitial is closed by the user, your OnNextLevel()
implementation will be called. (If the AppBrain interstitial has already been shown recently, it won’t be shown again and OnNextLevel()
will be called immediately.)
Other AppBrain Unity scripts
ExitInterstitial.cs
: Shows an AppBrain interstitial when the user exits your app. Simply attach this script to one of the main objects (for instance the camera) of your main scene, and you will automatically get the interstitial on back press.OfferWallButtonListener
: Attach this script to a Unity button, and set the parametermyButton
to the button. The AppBrain offer wall will then be opened when the button is clicked.
Calling AppBrain directly
The AppBrain SDK for Unity wraps most features from the AppBrain SDK in C# code. This means you can use the AppBrain SDK as described in the documentation, with only minor adjustments. For example, the following Java code:
AppBrain.getAdvertiserService().sendConversionEvent("iab", cents);
would in C# look like:
AppBrain.GetAdvertiserService().SendConversionEvent("iab", cents);
Note that AppBrain banners are currently not available from Unity. Feel free to contact us directly at contact@appbrain.com if you need any assistance.
Compilation tips
If you encounter errors when building your apps in Unity, please change the Build System setting to use Gradle.
In the Unity editor go to “Build Settings”, choose Android, then change the “Build System” option from “Internal (Default)” to “Gradle (New)”.
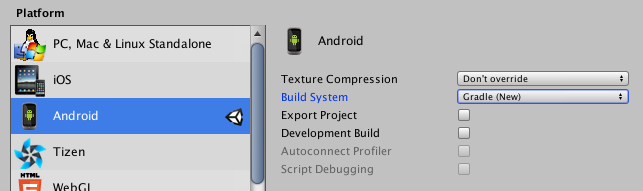